セットアップ
WordPress のプラグインとしてアプリケーションを構築します。このため、WordPress 本体のインストールが必要です。方法はさまざまですが、1つの方法として、コードによるコントリビューション入門 の指示に従ってください。セットアップの完了後は、このチュートリアルの残りの部分を進められます。
また、このチュートリアルでは、ステート、アクション、セレクタなど、Redux の概念に激しく依存します。もしそれらに馴染みがなければ、Getting Started With Redux の復習から始めるとよいでしょう。
プラグインの作成
開発はすべて WordPress のプラグイン内で行います。まず、ローカルの WordPress 環境に wp-content/plugins/my-first-gutenberg-app
ディレクトリを作成します。そのディレクトリの中に、4つのファイルを作成してください。
- my-first-gutenberg-app.php – 新しい管理ページの作成
- src/index.js – メインの JavaScript アプリケーション
- style.css – 最低限のスタイルシート
- package.json – ビルドプロセス用
以下のスニペットを使って、これらのファイルを作成してください。
src/index.js:
import { createRoot } from 'react-dom';
function MyFirstApp() {
return <span>Hello from JavaScript!</span>;
}
const root = createRoot( document.getElementById( 'my-first-gutenberg-app' ) );
window.addEventListener(
'load',
function () {
root.render(
<MyFirstApp />,
);
},
false
);
style.css:
.toplevel_page_my-first-gutenberg-app #wpcontent {
background: #fff;
height: 1000px;
}
button .components-spinner {
width: 15px;
height: 15px;
margin-top: 0;
margin-bottom: 0;
margin-left: 0;
}
.form-buttons {
display: flex;
}
.my-gutenberg-form .form-buttons {
margin-top: 20px;
margin-left: 1px;
}
.form-error {
color: #cc1818;
}
.form-buttons button {
margin-right: 4px;
}
.form-buttons .components-spinner {
margin-top: 0;
}
#my-first-gutenberg-app {
max-width: 500px;
}
#my-first-gutenberg-app ul,
#my-first-gutenberg-app ul li {
list-style-type: disc;
}
#my-first-gutenberg-app ul {
padding-left: 20px;
}
#my-first-gutenberg-app .components-search-control__input {
height: 36px;
margin-left: 0;
}
#my-first-gutenberg-app .list-controls {
display: flex;
width: 100%;
}
#my-first-gutenberg-app .list-controls .components-search-control {
flex-grow: 1;
margin-right: 8px;
}
my-first-gutenberg-app.php:
<?php
/**
* Plugin Name: My first Gutenberg App
*
*/
function my_admin_menu() {
// Create a new admin page for our app.
add_menu_page(
__( 'My first Gutenberg app', 'gutenberg' ),
__( 'My first Gutenberg app', 'gutenberg' ),
'manage_options',
'my-first-gutenberg-app',
function () {
echo '
<h2>Pages</h2>
<div id="my-first-gutenberg-app"></div>
';
},
'dashicons-schedule',
3
);
}
add_action( 'admin_menu', 'my_admin_menu' );
function load_custom_wp_admin_scripts( $hook ) {
// Load only on ?page=my-first-gutenberg-app.
if ( 'toplevel_page_my-first-gutenberg-app' !== $hook ) {
return;
}
// Load the required WordPress packages.
// Automatically load imported dependencies and assets version.
$asset_file = include plugin_dir_path( __FILE__ ) . 'build/index.asset.php';
// Enqueue CSS dependencies.
foreach ( $asset_file['dependencies'] as $style ) {
wp_enqueue_style( $style );
}
// Load our app.js.
wp_register_script(
'my-first-gutenberg-app',
plugins_url( 'build/index.js', __FILE__ ),
$asset_file['dependencies'],
$asset_file['version']
);
wp_enqueue_script( 'my-first-gutenberg-app' );
// Load our style.css.
wp_register_style(
'my-first-gutenberg-app',
plugins_url( 'style.css', __FILE__ ),
array(),
$asset_file['version']
);
wp_enqueue_style( 'my-first-gutenberg-app' );
}
add_action( 'admin_enqueue_scripts', 'load_custom_wp_admin_scripts' );
package.json:
{
"name": "09-code-data-basics-esnext",
"version": "1.1.0",
"private": true,
"description": "My first Gutenberg App",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
"keywords": [
"WordPress",
"block"
],
"homepage": "https://github.com/WordPress/gutenberg-examples/",
"repository": "git+https://github.com/WordPress/gutenberg-examples.git",
"bugs": {
"url": "https://github.com/WordPress/gutenberg-examples/issues"
},
"main": "build/index.js",
"devDependencies": {
"@wordpress/scripts": "^24.0.0"
},
"scripts": {
"build": "wp-scripts build",
"format": "wp-scripts format",
"lint:js": "wp-scripts lint-js",
"packages-update": "wp-scripts packages-update",
"start": "wp-scripts start"
}
}
ビルドパイプラインのセットアップ
このチュートリアルは、読者が ESNext の構文と、 webpack などのビルドツールの概念に慣れていることを前提とします。もし分からないところがあれば、まず、JavaScript ビルド環境のセットアップ を復習してください。
ビルドツールをインストールするには、ターミナルでプラグインのディレクトリに移動し、 npm install
を実行してください。
依存関係がすべて解決すれば、あとは npm start
を実行するだけで完成です。ターミナルで Watcher が実行されます。テキストエディタでコードを書くと、保存するたびに、自動的にビルドされます。
動作のテスト
管理画面の「プラグイン」を開くと、「My first Gutenberg App」プラグインが表示されているはずです。これを有効化すると、新しいメニュー項目「My first Gutenberg app」が表示されます。クリックすると、「Hello from JavaScript!」と書かれたページが表示されます。
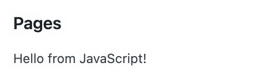
おめでとうございます ! これでアプリケーション構築の準備ができました !
次のステップ
- 前のステップ: はじめに
- 次のステップ: 簡単なページリストの構築
- (オプション) gutenberg-examples リポジトリ内の 完成したアプリ を参照
最終更新日: